Web Development with Blazor Framework
Modern web development technologies offer a wide range of tools and frameworks for creating dynamic and interactive applications. However, most of them are based on JavaScript, which requires developers to know at least two languages – JavaScript for frontend and C# or Java for backend.
Web Development with Blazor changes this approach by allowing to develop full-featured web applications in C#. In this section we will cover what is Blazor, what are its key advantages and why it is becoming more popular among .NET developers.
What Is Blazor?
Blazor is a framework from Microsoft for creating interactive web applications using C# and Razor components. It is based on .NET and allows writing a frontend without JavaScript, using WebAssembly or a server model.
Main Features of Blazor:
According to the Celadonsoft experience in MVP development with Blazor:
- Full C# on client and server – development of frontend and backend in the same language.
- Using Razor components is a convenient way to create UI.
- Interaction with JavaScript – possibility to use existing JS libraries if necessary.
- Support .NET-ecosystem – access to all ASP.NET capabilities and integration with existing services.
Blazor’s Advantages for Web Development
Why do developers from Celadonsoft choose Blazor instead of traditional JavaScript frameworks?
- Single stack – no need to switch between C# and JavaScript.
- High performance – WebAssembly allows running C# in the browser without interpretation.
- Code reuse – can be reused logic from server-based. NET applications.
- Deep integration with ASP.NET Core – simplifies the development of corporate web applications.
- Microsoft support – long-term development and stability of the framework.
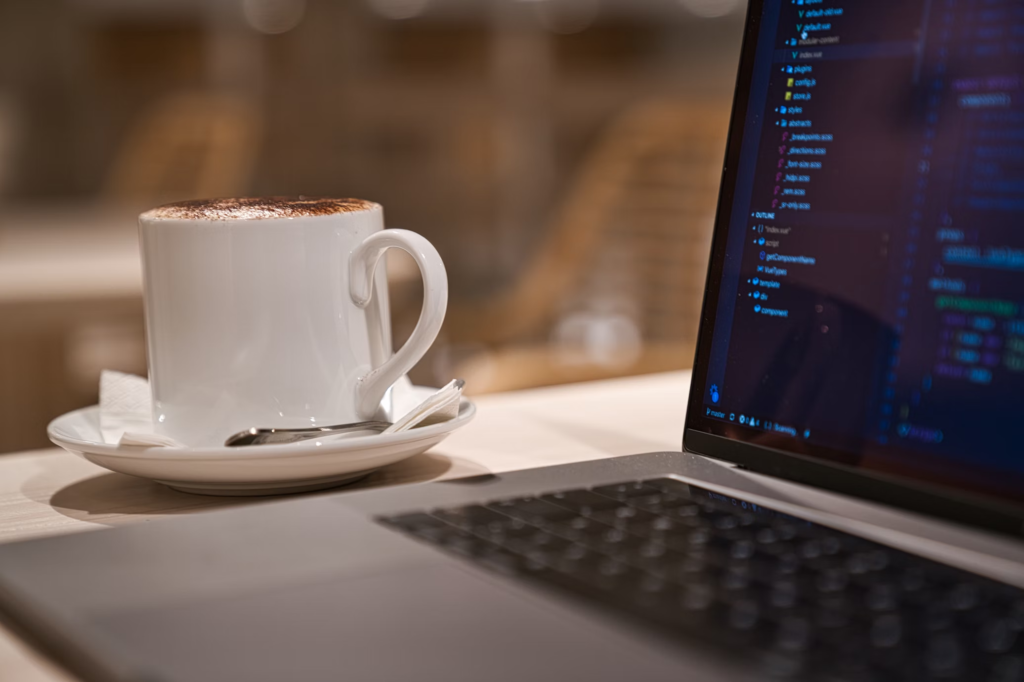
Architecture and Hosting Models of Blazor
Web Development with Blazor offers two main approaches to hosting applications: Blazor Server and Blazor WebAssembly. Both options allow for the development of interactive web applications using C# and .NET, but differ in architecture, performance, and application.
Blazor Server: Server Execution Model
In this approach, all calculations are performed on the server and user interface updates are passed to the browser via SignalR.
Key features:
- Instant launch – the code is executed on the server, and only HTML and minimal JavaScript are sent to the client-side.
- Minimal load on the client – no need to load all of .NET Runtime into the browser.
- Real-time feedback – thanks to WebSocket connection via SignalR.
Pros:
- Quick launch even on weak devices.
- Centralized code management and security.
- Ability to interact with server resources without additional API.
Cons:
- Delays in UI operation when the server is under high load or slow connection.
- Requires constant connection to the server.
- Higher load on the server when increasing the number of users.
Blazor WebAssembly: Client Execution Model
In this option, the application is loaded and executed directly in the browser using WebAssembly.
Key features:
- Full client execution – all code works on the client side, without the need for a constant connection to the server.
- Cross-platform – the application runs in any modern browser supporting WebAssembly.
- Native execution of C# in browser – no need to use JavaScript.
Pros:
- Work without a permanent connection to the server.
- High performance through local execution.
- Possibility of caching and offline mode.
Cons:
- Long boot time at first launch due to the need to boot .NET Runtime.
- Limited access to server resources without API.
- More memory consumption, especially in complex applications.
How to Choose the Right Model?
Blazor Server versus Blazor WebAssembly: That depends on the needs of your project:
- The Blazor Server is good to go for Corporate Applications, Dashboards, Systems with high data security.
- While Blazor WebAssembly is more suitable for a Standalone Web Application, SPA, and script with very negligible dependency on a server.
- Actually, there’s also a thing called Blazor Hybrid that provides the ability to run Blazor applications in Desktop and Mobile Environments-for example, with the.NET MAUI.
Blazor allows flexibility in choosing the right architecture, thereby assuring design convenience and support for one stack of technologies.
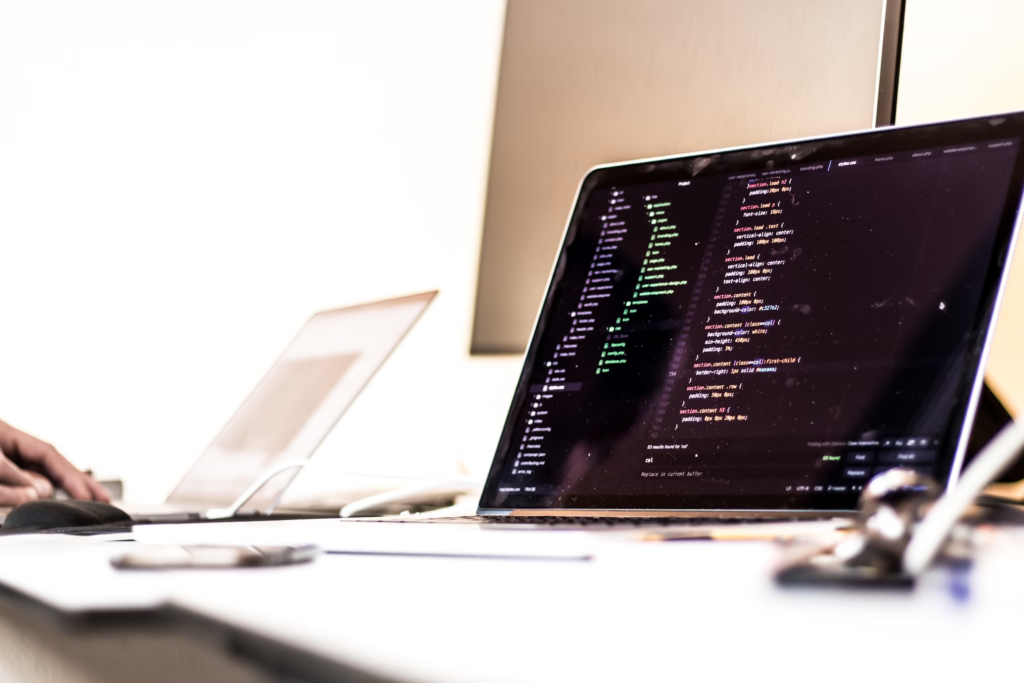
Getting Started with Blazor
Blazor is a powerful tool for Web Development with Blazor using C# that lets you make modern web applications, all without having to write JavaScript code. In this section, you will go through configuration of the development environment and your first application on Blazor.
1. Setting Up the Development Environment
To start working with Blazor, you need to install the following:
- .NET SDK – the most recent version of.NET is recommended. Use.NET 8 or later.
- IDE – the most comfortable option, Visual Studio 2022 (for Windows), or Visual Studio Code (for macOS and Linux).
- Blazor extensions – if you work in Visual Studio, during project creation, install the necessary components.
2. Creation of the First Blazor Project
When the tools are installed, you can create your first project.
Step 1. Create a new project
Open Visual Studio 2022 and select Create new project. In the search, specify Blazor and select one of the following templates: Blazor WebAssembly App – for client application (SPA). Blazor Server App – for server rendering. Please specify the project name and path to save.
Step 2. Project configuration
When creating a project, you can choose additional options: ASP.NET Core Hosted – allows creating a full-stack application with backup on ASP.NET Core.
Authentication – you can enable authentication support with Identity or with external providers at once.
Step 3. Run the app
Press F5 in Visual Studio to launch the project. By default, the application will be reachable at https://localhost:5001/.
3. Blazor-Project Structure
The Blazor project has the following structure:
- wwwroot/ – static files (CSS, JS, images).
- Pages/ – Razor-components (application pages).
- Shared/ – common components (such as navigation and header).
- Program.cs is the entry point, application configuration.
Each page in Blazor is a Razor component that contains layout (HTML) and logic (C#).
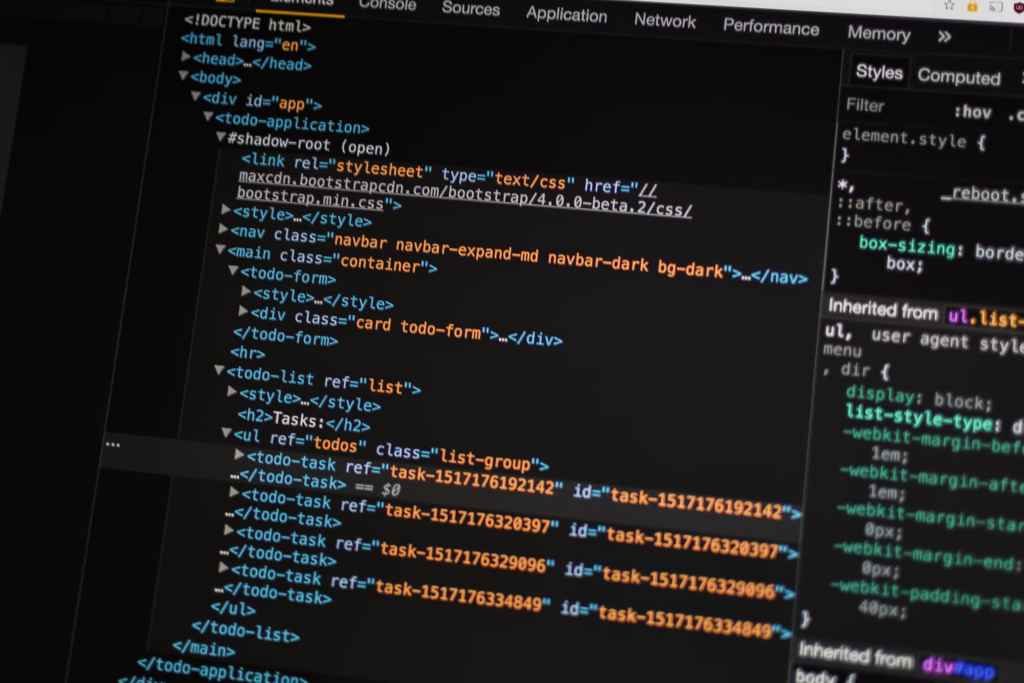
Creating Components in Blazor
Components are the building blocks of an application that enable you to share code by breaking it up into reusable, reusable pieces. This is the most flexible and easiest way to build your application.
1. What Are the Components in Blazor?
A Blazor component is a UI element, written using Razor syntax, that is, HTML + C#. Every component is a file with an extension of.razor, including:
- Markup (HTML)
- C# code-component logic
- Data and event interaction
2. Component Life Cycle
Blazor allows controlling the life cycle with following:
- OnInitialized- invoked in case of a component initialization
- OnParametersSet – Works if parameters changed
- OnAfterRender – run once UI is rendering
3. Creating and Reusing Components
This simple component can be done in the below way:
<h3>Hello, @Name! </h3>
@code {
[Parameter]
public string Name { get; set; }
}
This component accepts the Name parameter that can be passed from its parent component.
4. Nested and Dynamic Components
Blazor provides the embedding of components into each other and changes of their content with the help of RenderFragment. It’s useful when you need to create complex UI components, for example, model windows and tables.
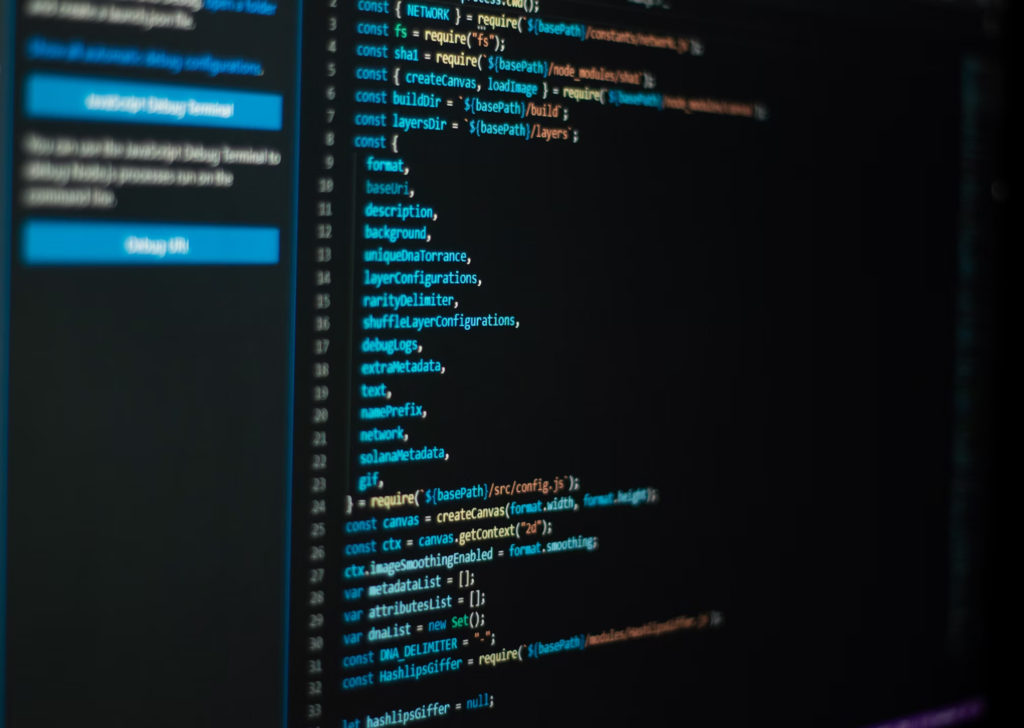
Status Management and Routing
To build convenient and scalable Blazor applications, it is important to properly organize routing and state management.
1. Routing to Blazor
Blazor uses a built-in routing mechanism that allows you to bind components to URL addresses:
@page "/about"
<h3>About us</h3>
Main routing capabilities:
- Setting parameters in URL (@page “/product/{id}”)
- Using NavLink for navigation
- Creating Custom Routes
2. Management of the State in Blazor
The application state can be managed in several ways:
- Inside the component (local state is used)
- Services (Singleton, Scoped) – allows storing and split data between components
- State Container (user services) – helps manage complex states
Example of global health through the service:
public class AppStateService
{
public string UserName { get; set; } = "Guest";
}
And then we use it in the component:
@inject AppStateService AppState
<h3>Hello, @AppState.UserName! </h3>
Working With Forms and Data Validation
Blazor offers a convenient form handling and built-in data validation support.
1. Creating Forms in Blazor
Forms are created using EditForm, InputText, InputNumber and other components:
<EditForm Model="@user">
<InputText @bind-Value="@user. Name" />
<button type="submit">Send</button>
</EditForm>
@code {
private User user = new User();
}
2. Data Validation
Blazor supports C# validation attributes:
public class User
{
[Required]
public string Name { get; set; }
}
To activate validation, you need to use DataAnnotationsValidator:
<EditForm Model="@user">
<DataAnnotationsValidator />
<ValidationSummary />
<InputText @bind-Value="@user. Name" />
</EditForm>
3. Event Processing and Data Sending
Blazor allows you to easily work with form events, for example sending data:
<EditForm Model="@user" OnValidSubmit="HandleSubmit">
<button type="submit">Save</button>
</EditForm>
@code {
private void HandleSubmit()
{
Console.WriteLine("Form sent!");
}
}
4. Working With External APIs and Validating on the Server
For complex validation scenarios, you can use server-side data verification via the API. For example, send data to the Web API, process it and return the result.
Integration With JavaScript and Third-Party Libraries
One of the advantages of Blazor is the possibility to interact with JavaScript, which enables you to use third-party libraries and plugins without losing the advantages of working with C#.
1. Blazor Interaction With JavaScript
Blazor features JavaScript Interop, which basically allows you to call any JS functions from your C# code and the opposite, really handy for integrating with UI libraries, and any sort of DOM manipulation and browser APIs in general.
2. Connecting to External Libraries
Blazor allows you to use popular libraries such as Chart.js, Leaflet, Bootstrap, and others.
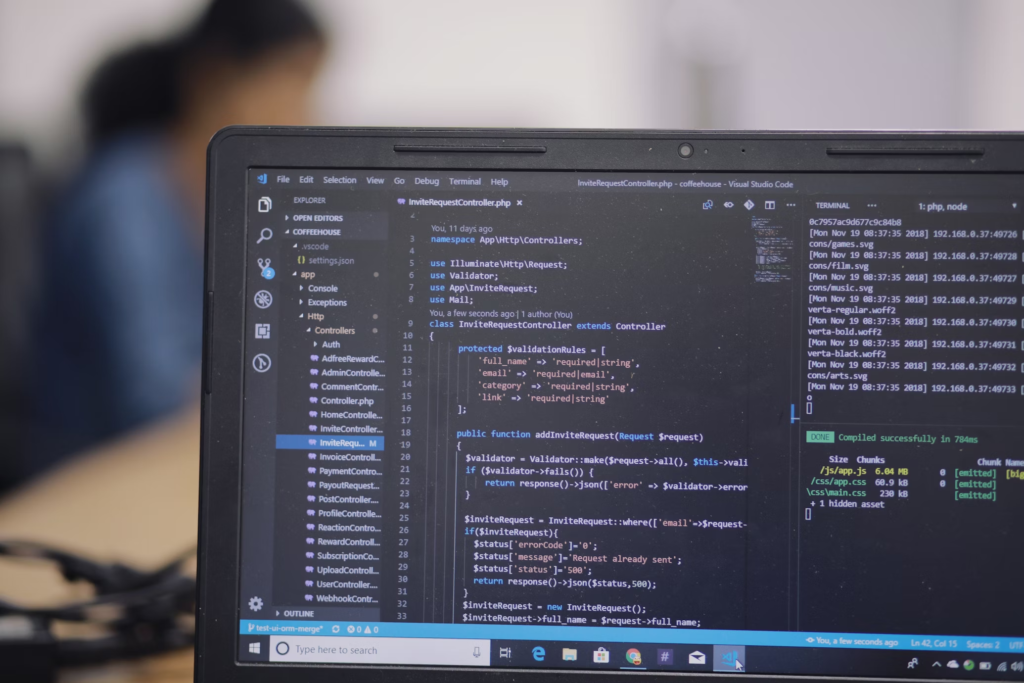
Optimization and Security of Blazor Applications
Blazor development requires not only writing code, but also performance optimization and data and user protection.
Optimization of Performance
While Blazor provides powerful tools, the following aspects should be considered:
- Lazy loading components – load only those parts of the application that are needed at the moment.
- Data optimization – minimize the number of HTTP requests and use caching.
- Resource compression – Reduce the size of JS/WASM files for faster boot times.
- Prefetch data (prerendering) to increase the speed of the first renderer.
Security at Blazor
Blazor supports modern methods of data and user protection:
- Authentication and authorization using ASP.NET Identity, OAuth and OpenID Connect.
- Protection from XSS and CSRF (Blazor automatically shields input, but be careful when using MarkupString).
- Use HTTPS for secure data exchange.
- Access roles and policies to restrict user rights.
Conclusion
Blazor web application development is a powerful and forward-looking approach that combines the capabilities of C# and .NET with web-friendly.
Why Choose Blazor?
- Full development in C# and .NET without the need to write JavaScript.
- Interactivity and support of the component approach.
- Built-in integration with ASP.NET, EF Core and other technologies.
- Possibility to use both Blazor Server and Blazor WebAssembly depending on project requirements.
Post Comment