Efficient Data Handling with React Query
In modern web applications, the need for effective data management is one of the key challenges. React Query is a library that greatly simplifies the work with server data, caching, queries and state synchronization. Celadonsoft will help us to consider the main features that make React Query a powerful tool for developers.
What Is React Query?
React Query is a tool for managing asynchronous queries in React MVP development services. It helps not only to execute server requests, but also to effectively cache, update and synchronize data in the application. Unlike standard approaches such as using useEffect and fetch, React Query provides a more convenient API for working with data, including:
- Automatic data caching, which reduces the load on the server.
- Support for real-time data updates.
- Convenient methods for handling boot, error, and success states.
- Tools for synchronizing data between components and keeping it up to date.
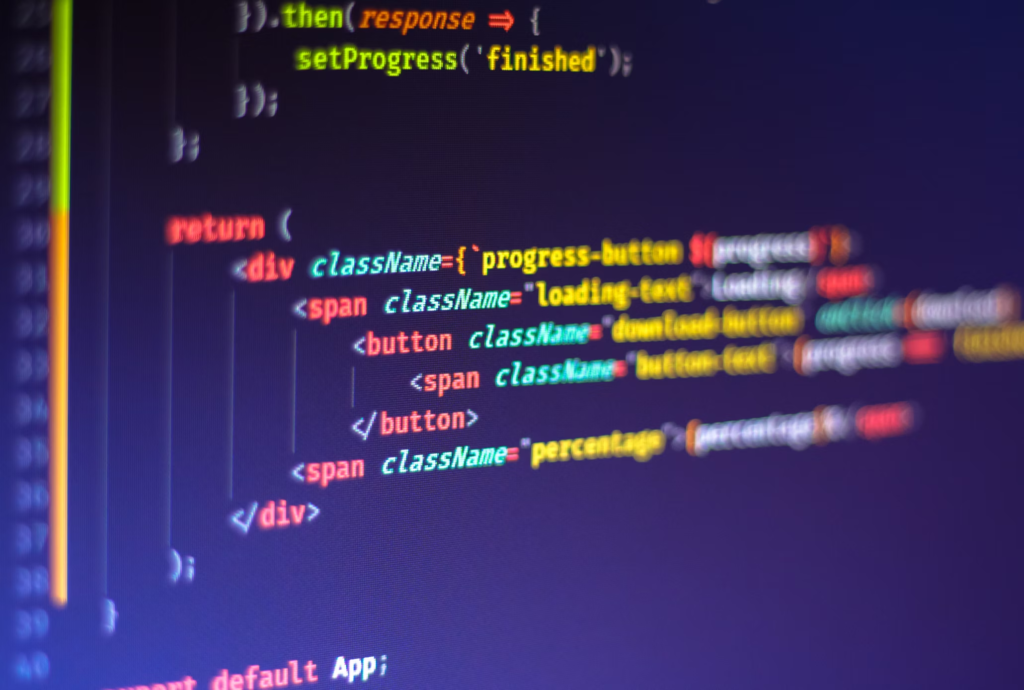
Why Use React Query?
Using React Query in a project greatly simplifies data handling and improves the performance of the fetching. Consider several reasons why this should be done:
- Reduce code duplication: React Query automates many processes, such as caching and re-querying, which reduces the amount of code that needs to be written by hand.
- Request State Management: The library offers standard mechanisms for handling boot states, errors and successful responses, eliminating the need for developers to manually monitor these states.
- Flexibility and scalability: React Query is well suited for applications of any complexity — from small to large projects with multiple API requests.
When Should I Use React Query?
React Query is recommended for use when your project requires large amounts of data, often updated from the server. This can be useful for such scenarios:
- Get data from server: If necessary, download the data from REST API or GraphQL.
- Real-time implementation: When the data on a client should be automatically updated when it changes on the server (for example, for chats, newsfeeds or dynamic lists).
- Interactive interfaces: For interaction with a server where data changes and must be synchronized between components (such as forms or dynamic tables).
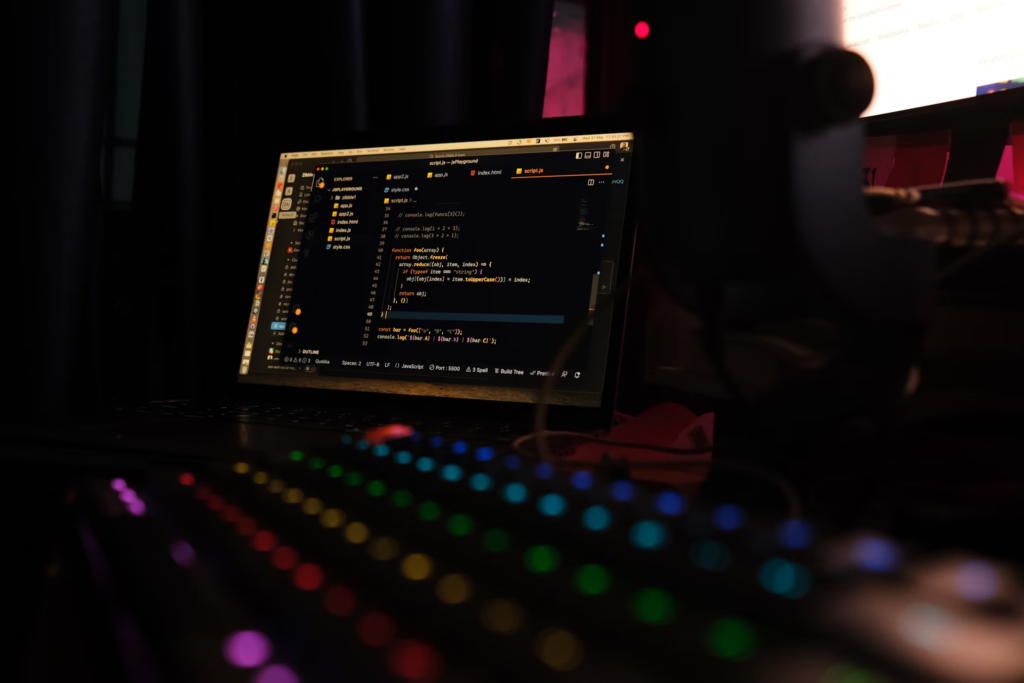
Basic React Query Hooks
React Query already provides a bunch of strong hooks that make your life with data much easier. Let’s touch upon their major features.
1. useQuery: Fetching Data from Server
useQuery is a hook, the basis of getting data in React applications using React Query. In fact, it really simplifies such aspects as executing asynchronous queries, caching, and refreshing data automatically. How is it working:
- Data fetching: useQuery hook allows you to request data from the server in an unproblematic way without taking care of the boot state and errors. It returns an object containing data, boot state, error, etc.
- Performance Optimization: React Query cached the results by default and avoided further unnecessary requests for the same resource. In return, it reduced the requests on the server side, optimizing the performance of the app.
Automatic update: Data can be updated automatically either at specified intervals of integration or when the data changes. Useful for applications with frequently changing data.
2. useMutation: Performing Data Mutations
Unlike useQuery, which is for fetching data, useMutation is for making changes — mutations, such as creating, updating, or deleting data on the server.
- Sending data to the server: You use useMutation when you want to do queries that alter the state on the server; for instance, when you’re adding a new element to the database.
- Processing errors and statuses: You can easily track if an operation has been successful or not, which in turn makes it easier to work with forms and other interface elements who change data needs to be processed.
Updater updates can be extended in enhancing user experience since change can instantly happen on the UI with an update function, maybe if the request at the server side is still waiting for something;
3.useQueryClient : To provide Access to Client Query Instance.
useQueryClient: This hook grants direct access, through an instance of React Query client, in cases when caching has to be managed in more flexible ways, or data need to be transformed-for example, on a mutation’s success.
- Caching: useQueryClient allows the independent updating and deletion of data in the cache. This may be necessary in particular after changing data so that the UI instantly shows the respective data.
- Query management: The hook also provides access to query management methods, such as canceling or restarting the queries, which enables operating data even more flexibly.
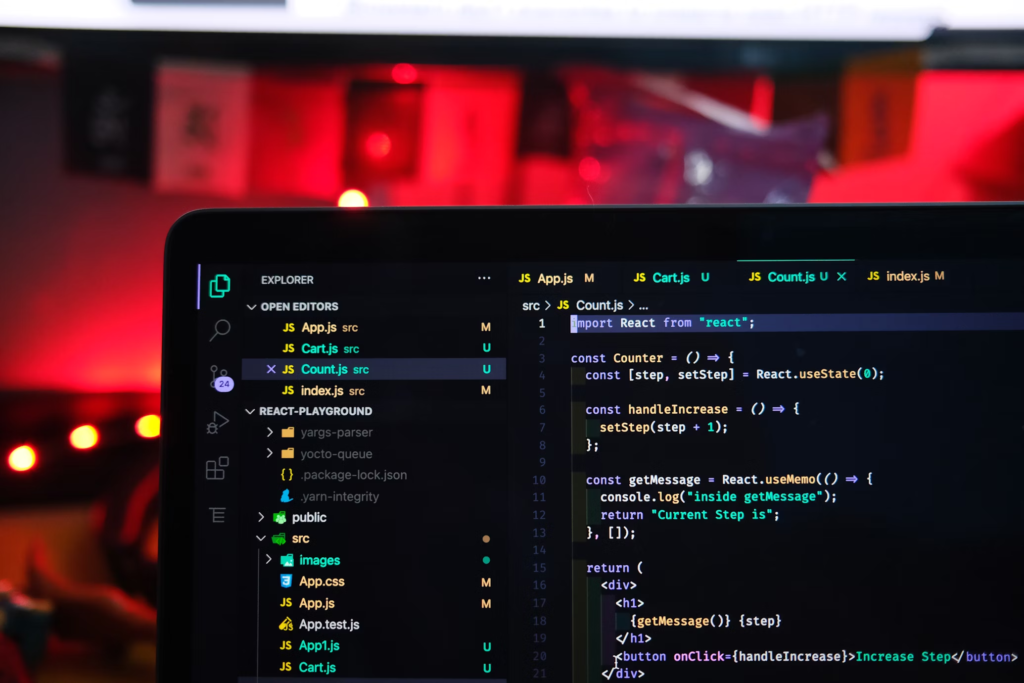
Caching and Automatic Updating of Data
React Query makes data state management much easier, and one of its key features is the effective caching of data and the automatic updating thereof. Consider how this works, and how configuration of these features can be done to increase your application’s performance.
Caching Data With React Query
Caching stands at the roots of React Query and is one of the most effective ways not to overload the server with too many requests. Once the data is loaded, React Query saves it in memory, and all the subsequent requests to this very data are executed much faster and without going to the server.
- Automatic caching: When you fire a query through useQuery, React Query by default caches the received data.
- Cache period: By default, the cached data stays in memory for 5 minutes. But this time can be configured using the staleTime option that allows avoiding repeated requests if data has not changed.
Cache Status Control
React Query provides rich possibilities for tuning the behavior of the cache:
- Cache depth: With the help of cacheTime, you are able to set how long the data will be cached. For example, increase this option if you want the data to stay longer.
- Forced cleaning of the cache: There are sometimes cases that require you to clean the cache manually. It is possible with queryClient.invalidateQueries or queryClient.removeQueries and thus manage application state.
The Advantages of Caching and Updating
- Reduced number of requests: the implementation of data caching reduces the load on the server, and because of the local storage of data, the application works faster.
- Productivity improvement: automatic updating allows you to keep data up-to-date without requiring users to manually update pages or retrieve information from it.
- Flexibility: with the help of settings, you can accurately specify when and how the data should be updated, which is already applicable to applications that require different freshness of information.
Error Handling and Boot State
To provide a great user experience working with remote data, proper error handling and boot state must be in place. React Query exposes convenient ways for both of those things.
Error Handling
With React Query, it’s easy to track errors for queries, by using an object returned from the useQuery hook by querying the isError property. If a request wasn’t successful, you can present a corresponding error message to your user.
Handling the Boot State
Having the isLoading property helps with tracking the state of the load of data-that might be extremely useful for displaying some sort of boot indicator inside your interface.
Optimistic Updates and Synchronization of Data
React Query is designed in such a way that it operates with optimistic updates, allowing for the interface to react faster from user events involving adding or deleting data without any need to await confirmation from a server.
An Optimistic Update-What?
React Query allows synchronizing data between components. It updates state automatically after requests or mutations. The useQueryClient hook permits access to a client instance in order to manipulate data directly on cache.
Data Synchronization
React Query allows synchronizing data between components. It updates state automatically after requests or mutations. The useQueryClient hook permits access to a client instance in order to manipulate data directly on cache.
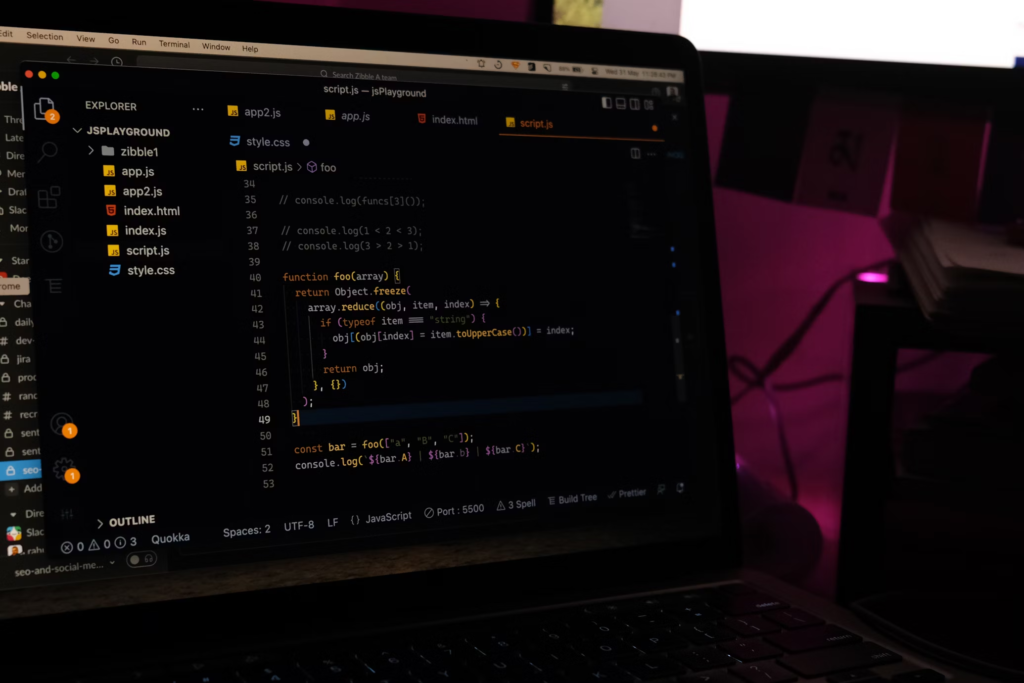
Best Practices and Tips
According to the Celadonsoft experience, to use React Query as effectively as possible, we recommend:
1. Use caching wisely
React Query automatically caches data, which avoids unnecessary requests to the server. However, it is worth paying attention to the cache lifetime settings, especially for data that changes frequently.
2. Update data in real time
Use automatic data update (refetchInterval) to keep the data in the application current without having to manually reload it.
3. Segment the requests by need
Never put all the queries into one. Segment them into smaller and isolated ones so that when you change one set of data, it doesn’t load the others.
4. Debug using DevTools
React Query DevTools is a very nice utility for seeing at one place the state of all the queries and mutations running in your app. It’ll give you insight into what’s cached, which requests are currently running, and some useful info on the status of the request.
5. Handle errors at the request level
Always think about the possibility of errors and implement some kind of error handling at the level of each request. This will help to improve the stability of the application.
Post Comment